Modern Calendar - How I made this project?
April 16, 2024
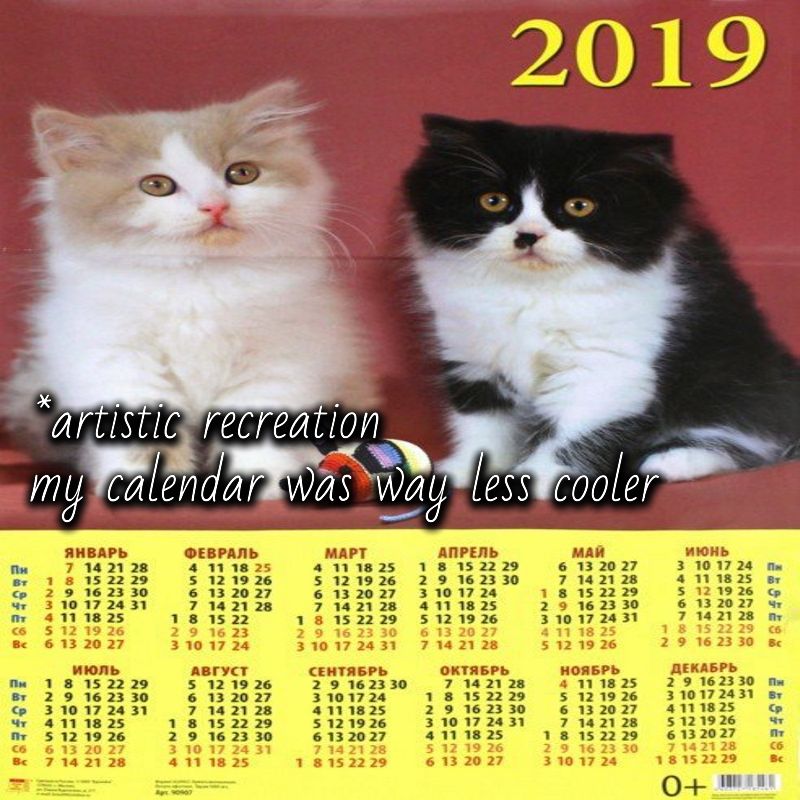
This text is a repost. Previously published on Personal site (July 6, 2019)
The idea to create my version of calendar was inspired by the video of popular YouTube channel Kurzgesagt. In that video they are talking about how humans should rethink their idea of timeline. They propose to change year number from 20xx to 120xx, so humans would always remember 10000 years of human progress.
I liked that idea and decided to learn more about different approaches to organizing days.
I dived into theme of different calendars and learned about International Fixed Calendar and then - perennial calendars, which can be applied to every year with same dates and weekdays.
Mix of perennial calendar and Kurzgesagt’s human era calendar was a fun idea and I decided to try write it as a browser app.
Originally, I wrote this code when I was taking my first JS course, so I was very excited to practice different methods with dates.
How converter should look?
My version of perennial calendar have just two simple rules:
- 28 days in a month
- Every week starts with monday
In this app I decided to start work from UI.
I wanted to keep it simple. One “card” for regular calendar, second “card” for converted one. On both should be date and week day.
Regular Calendar
This is the simple part. First, I needed to create today variable and get current date with Date() function.
let today = new Date();
And then just use a couple of methods to get values, that I need.
let regularDate, regularMonth, regularYear, dayArray;
regularDate = today.getDate();
regularMonth = today.getMonth() + 1; //Month starts with 0
regularYear = today.getFullYear();
Getting number of weekday is achievable with .getDay method. This number can be used as an index in array of days.
dayArray = ["Sunday", "Monday", "Tuesday", "Wednesday", "Thursday", "Friday", "Saturday"];
dayOfWeekOld = dayArray[today.getDay()]
The last step - is to assign this variables to the DOM.
regularDateDOM.innerHTML = regularDate + '.' + regularMonth + '.' + regularYear;
regularWeekdayDOM.innerHTML = dayOfWeekOld;
Modern Calendar
Conversion to Modern calendar is easy too, but it needs several calculations. Main value we should get is current day’s number. To calculate a day of a year we need to:
-
Subtract year (in milliseconds) from today (in milliseconds) - we will get how many milliseconds passed since a beginning of the year.
-
To calculate day we need to divide this number by 86400000 (1000ms * 60 seconds * 60 minutes * 24 hours).
The result will be how many days passed since a beginning of the year.
currentDay = Math.ceil((today - new Date(today.getFullYear(),0,1)) / 86400000);
Knowing this variable, we could calculate everything else.
To figure out the Modern Calendar's date, first we need to know how many full months passed. Full month could be calculated by dividing number of days by 28 (length of one month in Modern calendar). Then this number should be rounded to next largest whole number.
modernFullMonth = Math.floor(currentDay / 28);
Date - how many days left after subtracting months multiplied by 28 days.
modernDate = (currentDay - (modernFullMonth * 28));
However, we don’t count months this way. So actual month number is a number of whole month plus 1.
modernMonth = modernFullMonth + 1;
Last thing we need to figure out is a weekday. Because every month is the same, we know that every first day of a month is monday, second is tuesday, etc. So we could write simple if else function.
Getting day of the week. If it is a part of the array, then its index is greater than -1, then it's certain day of a week.
if ([1,8,15,22].indexOf(modernDate) > -1) {
modernDayOfWeek = "Monday"
} else if ([2,9,16,23].indexOf(modernDate) > -1) {
modernDayOfWeek = "Tuesday"
} else if ([3,10,17,24].indexOf(modernDate) > -1) {
modernDayOfWeek = "Wednesday"
} else if ([4,11,18,25].indexOf(modernDate) > -1) {
modernDayOfWeek = "Thursday"
} else if ([5,12,19,26].indexOf(modernDate) > -1) {
modernDayOfWeek = "Friday"
} else if ([6,13,20,27].indexOf(modernDate) > -1) {
modernDayOfWeek = "Saturday"
} else if ([7,14,21,28].indexOf(modernDate) > -1) {
modernDayOfWeek = "Sunday"
};
Then we assign every variable to the DOMs. As a result, we have one “card” for each calendar - one with standard values, one - with converted. In the end, I decided to add one more “card” with common values for both calendars - time and day of year.
Thanks for reading.